Node API
renderToFile
Helper function to render a PDF into a file.
Usage
const MyDocument = () => (
<Document>
<Page>
<Text>React-pdf</Text>
</Page>
</Document>
);
await renderToFile(<MyDocument />, `${__dirname}/my-doc.pdf`);
Arguments
Prop name | Description | Default |
---|---|---|
document | Document's root element to be rendered | undefined |
path | File system path where the document will be created | undefined |
callback | Function to be called after rendering is finished | undefined |
renderToString
Helper function to render a PDF into a string.
Usage
const MyDocument = () => (
<Document>
<Page>
<Text>React-pdf</Text>
</Page>
</Document>
);
const value = await renderToString(<MyDocument />);
Arguments
Prop name | Description | Default |
---|---|---|
document | Document's root element to be rendered | undefined |
Returns
String representation of PDF document
renderToStream
Helper function to render a PDF into a Node Stream.
Usage
const MyDocument = () => (
<Document>
<Page>
<Text>React-pdf</Text>
</Page>
</Document>
);
const stream = await renderToStream(<MyDocument />);
Arguments
Prop name | Description | Default |
---|---|---|
document | Document's root element to be rendered | undefined |
Returns
PDF document Stream
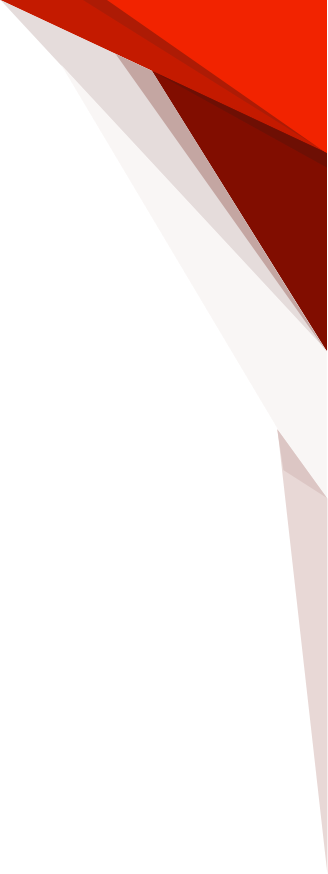